What is C language?
C is a general-purpose programming language created by Dennis Ritchie at the Bell Laboratories in 1972.
Table of Contents
It was mainly developed as a system programming language to write the operating system like as UNIX, WINDOWS, many other.
It is said that ‘C’ is a god’s programming language. One can say, C is a base for the programming. If you know ‘C,’ you can easily grasp the knowledge of the other programming languages that uses the concept of ‘C’.
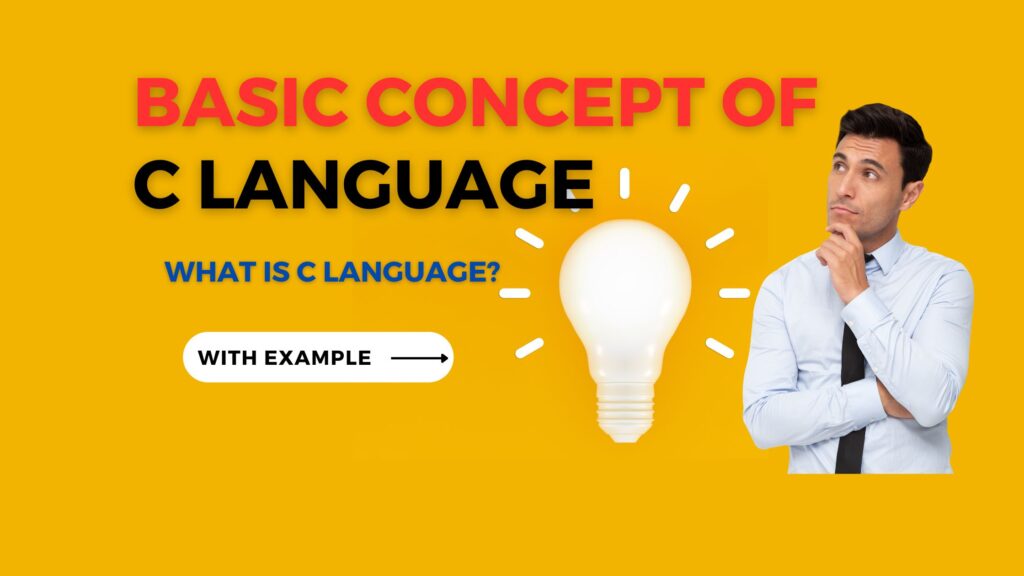
Main features of ‘C’ language:
- General Purpose and Portable
- Low-level Memory Access
- Fast Speed
- Clean Syntax
How ‘C’ Programming Language works?
The C programming language works by writing your code into an executable file. C is a compiled language. A compiler is a special tool that compiles the program and converts it into the object file which is machine readable. After the compilation process, the linker will combine different object files and creates a single executable file to run the program. The following diagram shows the execution of a ‘C’ program.
BEGINNING WITH C PROGRAMMING:
Write the first ‘C’ program:
#include <stdio.h>
int main()
{
int a = 10;
printf(“%d”, a);
return 0;
}
SOME PROGRAMS OF ‘C’ LANGUAGE:
Add two Numbers in C:
#include<stdio.h>
#include<conio.h>
int main()
{
int num1, num2, add;
printf(“Enter any two number: “);
scanf(“%d%d”, &num1, &num2);
add = num1+num2;
printf(“\nSum of %d and %d is %d”, num1, num2, add);
getch();
return 0;
}
C Program to Perform Add, Subtract, Multiply and Divide:
#include<stdio.h>
#include<conio.h>
int main()
{
int num1, num2, res;
printf(“Enter any two number: “);
scanf(“%d%d”, &num1, &num2);
res = num1+num2;
printf(“\nAddition = %d”, res);
res = num1-num2;
printf(“\nSubtraction = %d”, res);
res = num1*num2;
printf(“\nMultiplication = %d”, res);
res = num1/num2;
printf(“\nDivision = %d”, res);
getch();
return 0;
}
Area of Square Program in C
#include<stdio.h>
#include<conio.h>
int main()
{
float len, area;
printf(“Enter length of Square: “);
scanf(“%f”, &len);
area = len*len;
printf(“\nArea = %0.2f”, area);
getch();
return 0;
}
Fahrenheit to Celsius in C:
#include<stdio.h>
#include<conio.h>
int main()
{
float fahrenheit, celsius;
printf(“Enter Temperature Value (in Fahrenheit): “);
scanf(“%f”, &fahrenheit);
celsius = (fahrenheit-32)/1.8;
printf(“\nEquivalent Temperature (in Celsius) = %0.2f”,
celsius);
getch();
return 0;
}
Reverse a Number using for Loop
#include<stdio.h>
#include<conio.h>
int main()
{
int num, rev, rem;
printf(“Enter the Number: “);
scanf(“%d”, &num);
for(rev=0; num!=0; num=num/10)
{
rem = num%10;
rev = (rev*10)+rem;
}
printf(“\nReverse = %d”, rev);
getch();
return 0;
}
C Program to Swap Two Numbers:
#include<stdio.h>
#include<conio.h>
int main()
{
int num1, num2, temp;
printf(“Enter Two Numbers:-\n”);
printf(“First Number: “);
scanf(“%d”, &num1);
printf(“Second Number: “);
scanf(“%d”, &num2);
printf(“\nBefore Swap:\n”);
printf(“First Number = %d\tSecond Number = %d”, num1, num2);
temp = num1;
num1 = num2;
num2 = temp;
printf(“\n\nAfter Swap:\n”);
printf(“First Number = %d\tSecond Number = %d”, num1, num2);
getch();
return 0;
}
C Program to Find and Print Sum of all Digit of any N:
#include<stdio.h>
#include<conio.h>
int main()
{
int num, sum=0, rem;
printf(“Enter any number: “);
scanf(“%d”, &num);
while(num>0)
{
rem = num%10;
sum = sum + rem;
num = num/10;
}
printf(“\nSum of Digit = %d”, sum);
getch();
return 0;
}
C if-else & Loop Programs
Check Even or Odd in C
#include<stdio.h>
#include<conio.h>
int main()
{
int num;
printf(“Enter any number: “);
scanf(“%d”, &num);
if(num%2 == 0)
printf(“\nIt’s an even number.”);
else
printf(“\nIt’s an odd number.”);
getch();
return 0;
}
Check Prime Number or Not in C
#include<stdio.h>
#include<conio.h>
int main()
{
int num, i, count=0;
printf(“Enter a number: “);
scanf(“%d”, &num);
for(i=2; i<num; i++)
{
if(num%i == 0)
{
count++;
break;
}
}
if(count==0)
printf(“\nIt’s a prime number”);
else
printf(“\nIt’s not a prime number”);
getch();
return 0;
}
Find Largest of Three Numbers using if-else-if:
#include<stdio.h>
#include<conio.h>
int main()
{
int a, b, c, big;
printf(“Enter three numbers: “);
scanf(“%d%d%d”, &a, &b, &c);
if(a>b)
{
if(b>c)
big = a;
else
{
if(c>a)
big = c;
else
big = a;
}
}
else
{
if(b>c)
big = b;
else
big = c;
}
printf(“\nLargest number = %d”, big);
getch();
return 0;
}
Find Factorial of Given Number in C:
#include<stdio.h>
#include<conio.h>
int main()
{
int num, i, fact=1;
printf(“Enter any number: “);
scanf(“%d”, &num);
for(i=num; i>0; i–)
fact = fact*i;
printf(“\nFactorial of %d = %d”, num, fact);
getch();
return 0;
}